Python 如何向csv文件添加时间戳
CSV文件是一种简单且广泛使用的格式,用于存储和交换数据,有时我们可能需要向CSV文件添加时间戳,例如当我们想要跟踪数据获取或更新的时间时。在本文中,我们将探讨如何在Python中向CSV文件添加时间戳。我们将讨论不同的添加时间戳的方法,如使用datetime模块和pandas库。我们还将提供逐步说明和代码示例,帮助您开始向CSV文件添加时间戳。
DateTime模块
DateTime模块可用于处理日期和时间,因为Python中没有日期或时间数据类型。Python已经内置了DateTime模块,因此我们不需要单独安装它。
Python DateTime模块提供了日期和时间操作类。在这些框架中,日期、时间和时间段是方法中的主要参数。因为在Python中Date和DateTime是对象而不是文本或时间戳,所以对它们进行的任何修改都会产生相同的效果。
Python的CSV模块
Python的csv模块可用于处理逗号分隔值(CSV)文件,这是一种常见的存储表格数据的方式。该模块具有读取和写入CSV文件、解析CSV数据和操作CSV数据的方法。
csv模块中有类可用于写入和读取以CSV格式呈现的表格形式数据。它允许程序员说出“以Excel所需的格式写入这些数据”或“从Excel生成的文件读取数据”,而无需知道Excel使用的CSV格式的确切细节。用户还可以描述其他应用程序可以理解的CSV格式,或者为特定目的创建自己的CSV格式。
csv模块的writer和reader对象用于读取和写入序列。使用DictReader和DictWriter类,程序员还可以以字典形式读取和写入数据。
向Python中的新CSV文件添加时间戳
添加时间戳到新文件的步骤
- 导入datetime和CSV模块。csv模块将用于接收和写入csv文件,而datetime模块将用于将当前日期和时间追加到csv文件中。
-
从用户获取要添加到文件中的数据。
-
使用open()函数以读写模式(’r+’)打开CSV文件。
-
open()函数打开文件并返回表示文件的文件对象。
-
通用换行模式的工作方式由换行符=“”设置控制。它可以是None、”,” “n,” “r,”或”rn”。
-
write()返回一个writer对象,负责将用户的数据转换成带有空格的字符串。
-
使用datetime获取当前日期和时间。
-
datetime模块有一个称为now()的函数。
-
借助for循环,遍历rows变量中的所有数据。
-
使用insert()函数,可以将当前日期和时间放置在每个数据的第0个索引处。
-
使用当前日期和时间,使用writerow()将数据写入CSV文件。
示例
# Importing all the important required modules
import csv
from datetime import datetime
# We will be storing data in the variable r
# After that we will add this data to our CSV file
r = [['Tutorialspoint', '--'],
['Simply', 'Easy'],
['learning', 'at fingertips']]
# Use open() module to open the CSV file
# open csv file in read and write mode
with open(r'C:/Users/Tutorialspoint/Desktop/sample2.csv', 'r+', newline='') as f:
# create the csv writer
fwrite = csv.writer(f)
# Now we will store current time and date
current_dnt = datetime.now()
# Iterating over each and every data in the r variable
for v in r:
# Inserting the date and time at 0th
# index
v.insert(0, current_dnt)
# writing the data in csv file
fwrite.writerow(v)
输出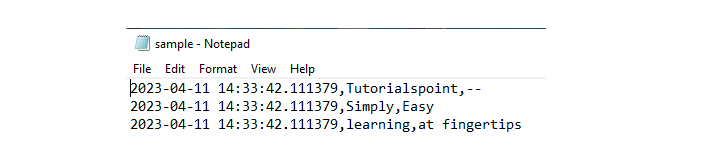
在Python中为现有的CSV文件添加时间戳
首先,以读取模式打开已经存在的文件和新文件。使用CSV模块的reader()函数将第一个文件转换为CSV reader对象。reader()函数会返回一个可以遍历CSV文件中每一行的对象。
使用for循环将第一个文件中的所有信息添加到rows变量中。使用CSV模块的writer()函数为第二个文件创建一个writer对象。然后,使用for循环遍历rows变量中的所有数据。将当前日期和时间存储在一个变量中。然后,使用insert()函数将其放置在数据的第0个索引位置。使用CSV模块的writerow()函数将保存在File2中的数据写入。
示例
import csv
from datetime import datetime
# We will create a list where we can store content of the file
r = []
# open the existing csv file in the read mode
with open(r'C:/Users/Tutorialspoint/Desktop/industry.csv', 'r', newline='') as f:
# Opening new CSV file to in write mode
# to add the data
with open(r'C:/Users/Tutorialspoint/Desktop/sample.csv', 'w', newline='') as f2:
# creating the csv reader
reader = csv.reader(f, delimiter=',')
# we will store the data of existing foile in r
for row in reader:
r.append(row)
# creating the csv writer
f_write = csv.writer(f2)
# Iterating over all the data in the r variable
for v in r:
# storing current date and time in a variable
current_dt = datetime.now()
v.insert(0, current_dt)
# writing the data in csv file
f_write.writerow(v)
输出
结论
在本文中,我们学到了一个叫做datetime的模块可以用来处理日期和时间,我们还可以使用CSV模块来操作CSV文件。我们已经看到了如何使用Python向新文件或现有文件添加时间戳。